My journey with React started more than a year ago. I was already doing web-development with HTML, CSS, and JavaScript for the past seven years. Later, I completed the Freecodecamp front end certification, too. React came like a cool kid in front end development, which was a must at the time. So, I jumped into the bandwagon and purchased "Modern React with Redux" by Stephen Grider and learnt a lot from it. Additionally, I learned a lot from React for beginners by Wes Bos. I highly recommend both the courses and this article is based on what I learned from it and my past nine months as a React developer for my company.
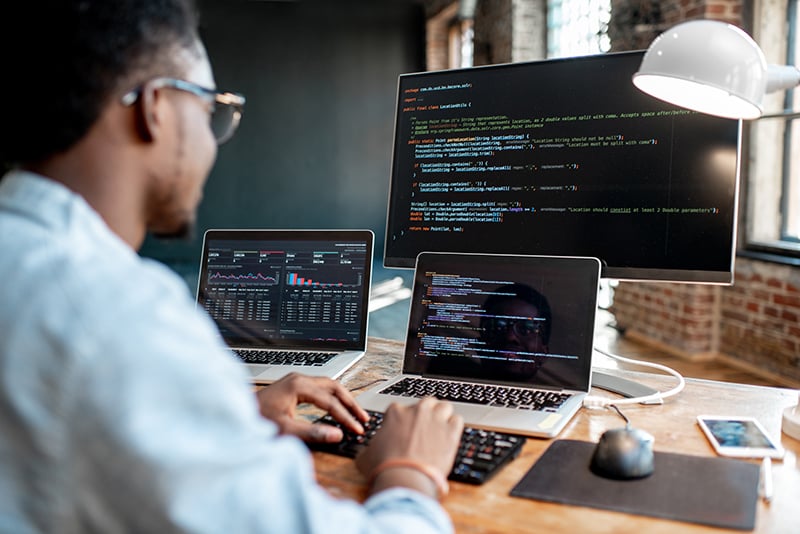
In React, we have to divide our project in small components. Later, we write those components and stick it into the main app component. As we are making a YouTube player app in React, we design the layout and create components for different parts.
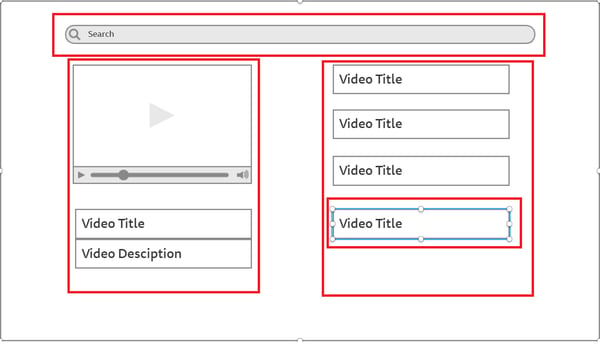
Wireframe with Components in RED
As shown in the above diagram, we have divided the app into four components — Search Bar, Video Player, Video List and Video List Items.
We will create React app using the awesome create-React-app from Facebook. Search for it, depending on your npm version, you have to follow different process.
For npm 5.1 and earlier and for npm 5.2 and up
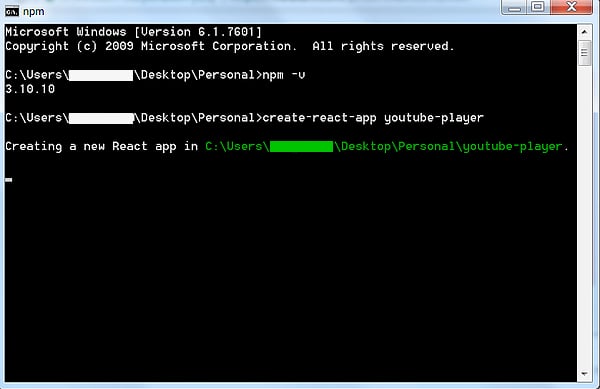
create-react-app
It will take 2–3 minutes to create the basis boilerplate app. CD into it and do “npm start”
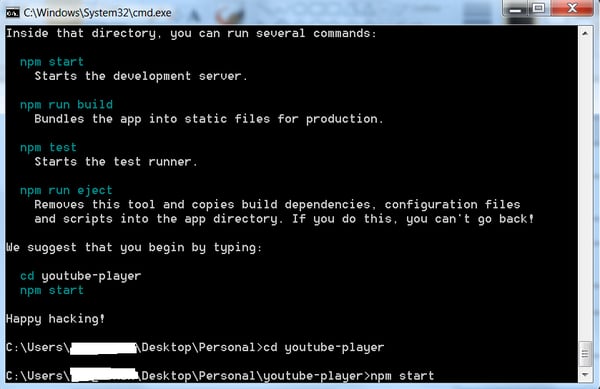
cd into it and npm start
It will start the app on http://localhost:3000/ and show the basic boilerplate, which we will change soon.
Basic boilerplate
The index.js renders all these from App.js and show it in the “root” in index.html element by - ReactDOM.render(<App />, document.getElementById(‘root’));
So, we will start our coding from App.js, which is our main component that is holding the other components mentioned earlier like Search Bar, Video Player, etc.
Open the folder youtube-player using your favourite code editor. I am using VSCode.
Update App.js as shown below.
import React, { Component } from ‘react’;
class App extends Component {
render() {
return (
Youtube App
);
}
}
export default App;
Create a new folder called Components inside src and create four files for our four components in it.
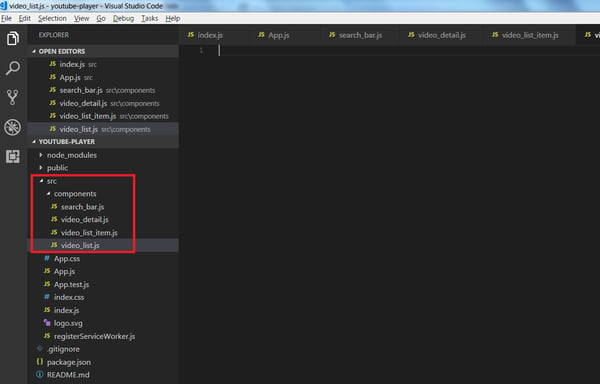
Creating files
As we will add YouTube to our app, head over to https://console.developers.google.com , to get the API keys.
Search for YouTube and then select YouTube Data API v3.
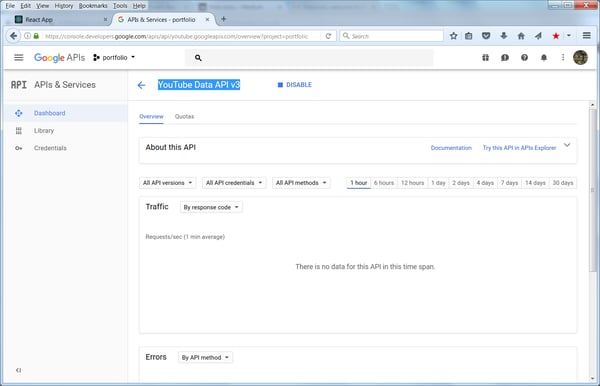
YouTube Data API v3
You have to “Enable API” and then head to Credentials and click “Create Credentials->API Key”
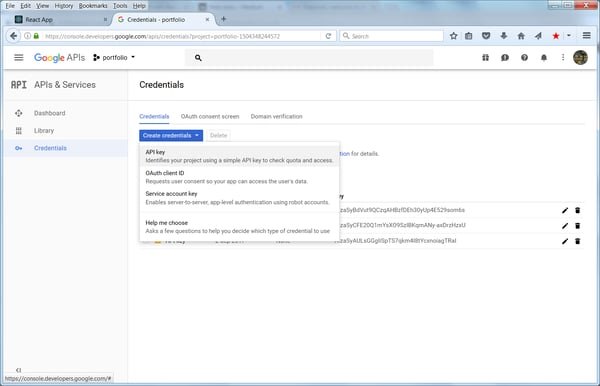
Create Credentials->API Key
Copy the API key to your App.js
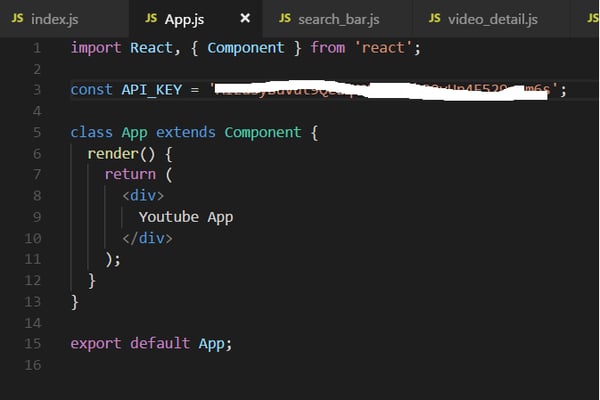
Copy the API key
We will install a package called youtube-api-search to help in searching. So head over to your command prompt and install in project through npm by.
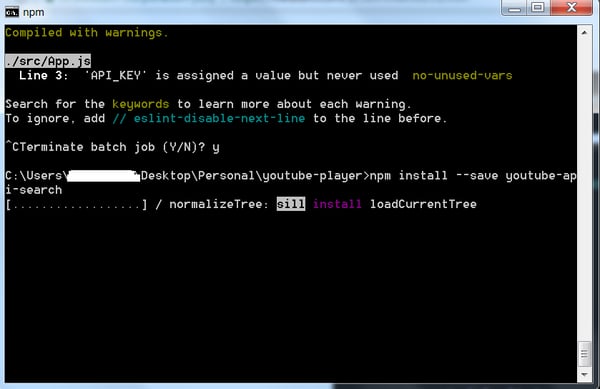
npm install — save youtube-api-search
Now, we will start creating our Search Bar component. Head over to it and change as shown below to create a class based component.
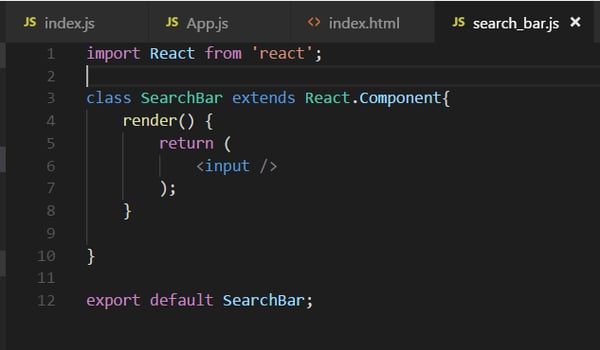
search_bar.js
Now, add this component to our App.js by adding the below lines.
How to Make YouTube Player in ReactJS image-2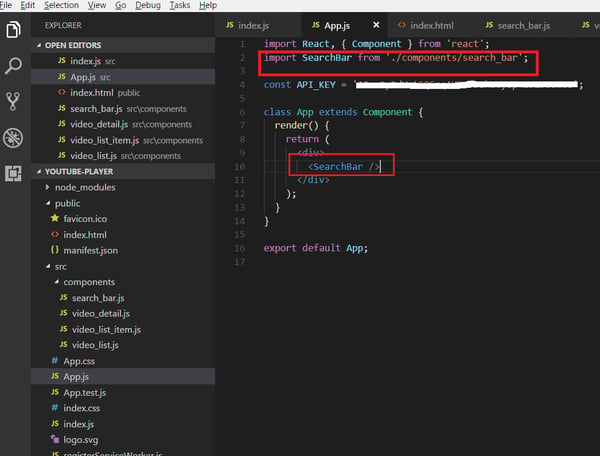
import SearchBar and display it.
Head over to React-app. It shows the input box. You should have npm start running for it.
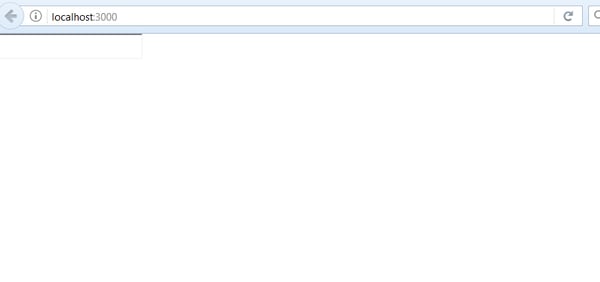
Showing input box
Now, we will complete the input field in our search_bar.js and it will have some new logic, which I will explain.
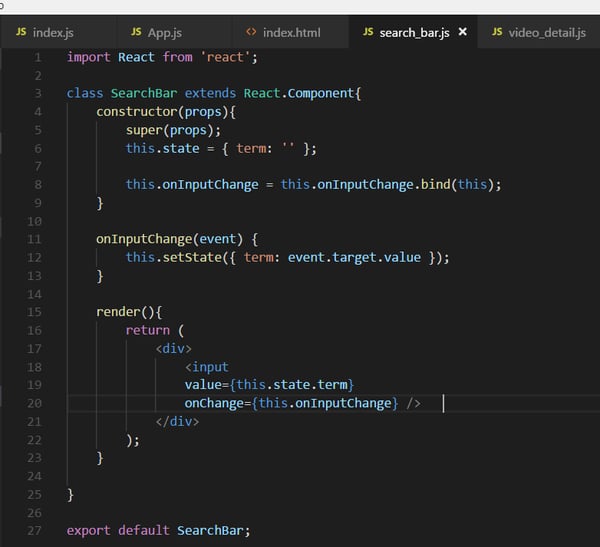
With state and on Change
We are using state, which is one of the key concept of React. It is an object of this file, which holds various key-value pairs. It is initialized in constructor by statement like this.state = { };
But when we need to change it, we can achieve it only by statement like this.setState({ }).
In React, whenever the state is changed, the render function is run again.
Now, let’s go through the flow to understand how state and input box works. We are using ES6 constructor, which will first run when the Search Bar is called first from index.js . Inside Constructor, we are setting our initial state object by this.state = { term: ‘’ }, which set a key-value pair. The value for key term is empty string. Ignore the next line; will come back to it later.
After the Constructor is run, it’s the turn of render() to run. It has an input field for which, value={this.state.term}, so we are setting it to value of term. This is empty string as value to term is ‘’. Notice that we are using {} because that’s how we show JSX inside our file.
We have an onChange={this.onInputChange}. This is a change handler but in React we add on to it. Other handlers are onClick, onFocus, etc.
Now, this onChange handler will wait until the user types something in input field. Suppose the user types ‘a’ in input box. It will trigger the function onInputChange.
Inside onInputChange we are using this.setState({ term: event.target.value }). It is changing the state object and setting term equal to event.target.value, which is a plain HTML event that triggered it. It is ‘a’ in this case, so term ‘a’.
Now, in React when we change the state the render() runs again. So, it will run and set the value in input with value={this.state.term} . Now, the value is ‘a’ for the key term.
This step will repeat every time we hit add an alphabet to input field and term gets updated to it.
One last thing. The line this.onInputChange = this.onInputChange.bind(this); in Constructor is required, or else in onChange where we are using this.onInputChange it won’t recognize it. The statement means we are binding this value to onInputChange
Hope, this article is helpful.
Read More: Create YouTube Player in ReactJs — Part 2
Read More: Create YouTube Player in ReactJs — Part 3
About Innominds
Innominds is a leading Digital Transformation and Product Engineering company headquartered in San Jose, CA. It offers co-creation services to enterprises for building solutions utilizing digital technologies focused on Devices, Apps, and Analytics. Innominds builds better outcomes securely for its clients through reliable advanced technologies like IoT, Blockchain, Big Data, Artificial Intelligence, DevOps and Enterprise Mobility among others. From idea to commercialization, we strive to build convergent solutions that help our clients grow their business and realize their market vision.
To know more about our offerings, please write to marketing@innominds.com